We Created A New Scripting Language for DeFi
Today we will take a look at our very own scripting language, created to simplify complex operations and open up on-chain scripting outside of Solidity.
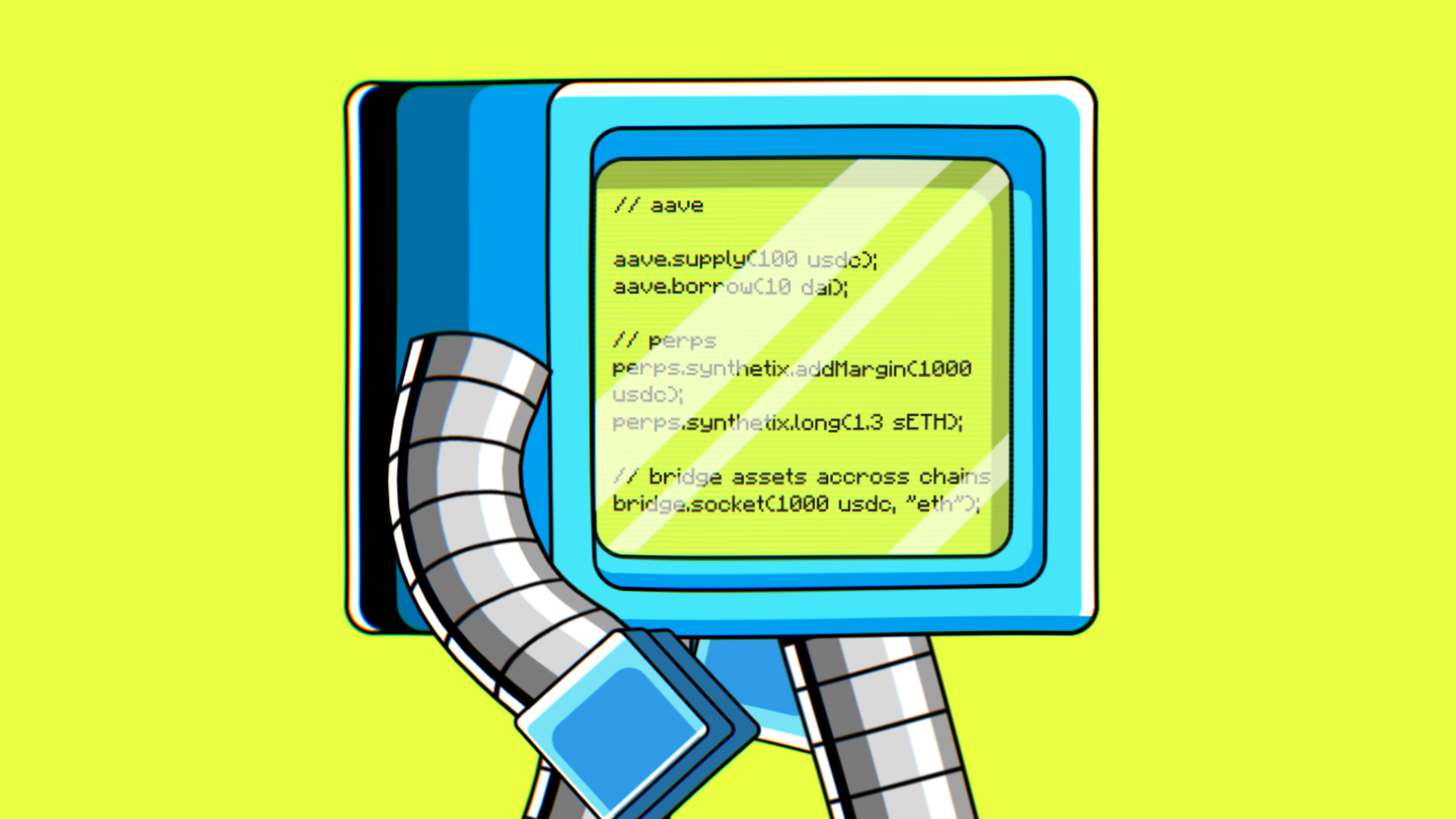
Welcome to the second in our series of technical articles exploring the inner workings of the Mass app and what makes it so unique. Today we will take a look at our very own scripting language: Mass Simplified Language (MSL). It was created to simplify complex operations and open up on-chain scripting outside of Solidity.
TLDR
We're addressing what we believe is a lack in the ecosystem: An entry-level way of scripting on-chain complex interactions.
Stay tuned and follow @MassDotMoney: we'll release our playground for you to experiment with the Mass Simplified Language (MSL) in a matter of days!
Why Create a New Scripting Language?
This year, the DeFi ecosystem has become very dynamic around things related to interoperability and complex interactions. There are a lot of shiny new things to play with, and the "what" is being well addressed in various innovative solutions across the ecosystem.
That said, I personally feel like this translates into a steady increase in complexity when it comes to the "how".
There were days when integrating a protocol was as simple as calling a Solidity interface. We now have more and more ways of interacting with different protocols (ERC721 signatures, asynchronous orders, off-chain APIs to prepare interactions, ...), all requiring developers to learn something radically different every time.
At Mass, our feeling is that while this dramatically improves our horizons, this also slows down quite a bit the pace at which developers can reason about their code. We see an ever increasing amount of complexity in smart-contracts, which limits our agility as developers.
But even more importantly, this prohibits beginners from using all those new tools without facing any doubt on the robustness of what they do, or without drowning in heterogenous documentation.
Let's also not mention the fact that Solidity is (arguably) a really shitty language to begin with. There are so many hiccups and traps to fall into.
We could also say the same of contract development as a means to write single-usage interactions, for that matter.
So, what's missing?
We have actually already seen a similar situation.
Way back in the day, the common way to build programs was to spend a day installing some tooling, writing some code, and compiling it before running it.
Then came scripting languages.
They greatly simplified the lives of people who know basic coding, but are not willing to write full fledged programs just to perform a simple task.
We believe that's what is missing. We need a more simple, safe and intuitive scripting language to write complex on-chain interactions between common well-known protocols. Solidity is simply a terrible option for this use case.
What We Built
We've built a scripting language that aims at:
- Providing a way for everyone (not only Solidity devs) to write on-chain scripts.
- Abstracting away implementation details (like ERC20 digits)
- Writing scripts that interact both with on-chain & off-chain APIs, seamlessly
- Protect users against mistakes or protocol misuses
- Protect users against copying/pasting code snippets that could lead to a theft of funds
- Adding support for things that are not supported by Solidity (such as decimal numbers)
- Having a much better code/compile/simulate/debug feedback loop
- Providing transparency on transactions with built-in decompilation (more on that later)
Examples can be worth a thousand words, so here are some concrete things that you will be able to write.
Some examples
Arithmetic & logic. Note how all values have a unit attached, and how the language abstracts decimal-related logic for your:
a = 1.2 eth;
b = 3.4 usdc;
x = a + b; // compile error ! cannot add eth & usdc
price = a / b; // this is ok, it is a price, in [usdc/eth]
// condition
if (price > 1234.56 [usdc/eth]) {
// do something
}
Execute multiple swaps, in one transaction:
// deposit funds from your wallet
vault.deposit(150 usdc);
// perform a USDC -> DAI swap on paraswap, with 3% slippage limit
daiResult = dex.paraswap(50 usdc, $dai, 3%);
log("received dai", daiResult);
// puts all dexes aggregators in competition
// to execute this swap (winner will be chosen by the compiler)
ethResult = dex.all(50 usdc, $eth, 3%);
// swap received eth direcly via a liquidity pool
// (with 5% slippage compared with coingecko price)
minResult = ethResult * prices.coingecko($eth, $btc) * 0.95;
usdtResult = dex.uniswapV2(ethResult, minResult);
log("received btc", usdtResult);
Interact with many protocols, in a single transaction:
// aave
aave.supply(100 usdc);
aave.borrow(10 dai);
// perps
perps.synthetix.addMargin(1000 usdc);
perps.synthetix.long(1.3 sETH);
// bridge assets accross chains
bridge.socket(1000 usdc, "eth");
// ... etc !
(coming soon) Schedule transactions for later:
// execute instructions after a given date
when now() > '2024-01-01 12:34'
with gas limit 1 usdc {
// ... instructions
}
// execute instructions when an oracle feed reaches a given price
when (prices.chainlink($usdc, $eth) > 1.23 [usdc/eth])
with gas limit 1 usdc {
// ... instructions
}
Send a transaction without any native tokens in your wallet (gas fees will be paid by using the token of your choosing):
// gas limit
#gasless 1 usdc;
// ... instructions
How and Where to Run Scripts
Our (new) security model
The gist is simple: anyone can ask to create one or many MSAs (Mass Smart Account).
An MSA is a smart contract that the "MSA factory" will deploy for you, over which you will have full control.
It can hold your funds, and you (and only you) can send instructions to it to manipulate them.
Those "instructions" are actually EVM instructions (in other words: code), which are dynamically executed by the HyVM.
If you follow us closely, you might have heard about the HyVM. If not, you can read about it in this article or read this X thread. In short, the HyVM enables you to execute on-chain dynamic scripts (which you can compile from EVM-languages like Solidity, Vyper, Huff...) without having to deploy a new contract.
So basically, you could spawn an editor, write some Solidity code, compile it, and send it for execution in the context of your MSA. No deployment is required, no gas fees associated with creating a contract, no approval, and no on-chain artifacts that will be there forever.
Each MSA allows you to not only send instructions to it directly, but also supports signing those instructions using ERC-712 if you want someone else to send it for your later. In other words: gasless transactions, and transaction scheduling.
It also supports signing multiple actions with a single wallet signature, thus enabling things like scheduling multiple actions at once (grid trading, ...).
Decentralisation & Upgradeability
Contract upgradability breaks decentralization, in a sense. If you don’t have the choice to upgrade as a user, you're subject to the will of protocol developers without being able to do much about it. But non upgradable contracts can also be broken in various ways.
However, there is a middle ground: On-demand upgradability.
You being the sole owner of your MSA enables us to let you choose whether or not to upgrade.
You can choose to upgrade and benefit from the new features we develop as we deploy them. But you can also choose not to upgrade them and stick with an older version. Or even to switch to a completely new implementation that has not been written by us.
Your funds, your MSA, your choice. That ensures you that Mass cannot in any way fiddle with your ability to stay in control of your funds.
A Word on Transparency: Decompilation
Because we believe transparency and verifiability are fundamental to the ecosystem, we ensured that the compiler of our scripting language is fully reversible.
This means that you can receive call data to send on-chain from a script (that's a classic compilation), but the inverse is also true! When you see on-chain call data, you can use our SDK to decompile it and get a script back from it (either in its string form or as an Abstract Syntax Tree).
This can have a profound impact in the long run.
- Every transaction is transparent
- We hope that someday, wallet developers will integrate our SDK to decompile the payloads that are sent to them when a user signs a transaction. By doing this, they could bring an unparalleled level of verifiability to what the user is currently signing.
- Keepers that will execute scheduled transactions can easily decompile transactions they intend to execute, and thus analyze in a structured way what the transaction is doing to better understand when to schedule it. This will be far superior compared to the current brute-force simulations.
- A complex transaction can look like a messy pile pretty fast, if you only look at tx logs. This makes the task difficult for DeFi front-ends to infer what a user has done and display it as a nice transaction history. Having a structured way to analyze MSA transactions can help them in many ways.
How to use the MSL?
Our playground & SDK
You will be able to use the Mass Simplified Language directly in our playground, which we will release in a couple of days. Subscribe to @MassDotMoney to get the news asap!
But you may also be interested in using it as a building block for your own product.
If this is the case, hang tight! We will also be releasing a stable version of our SDK soon, which is basically a compiler that translates code into instructions to execute (a transaction to sign using ERC721, or to send on-chain).
In the meantime, we at Mass will be building utilizing everything mentioned above to bring DeFi the front-end that we believe it deserves.
The future
We'd like to integrate as many protocols as we can into our SDK, allowing users to have a single, simple, and homogenous way of interacting with them.
If you're a protocol builder, and would like to see your name in our scripts, please contact us via our Discord server.
We'll also expand the capabilities of our Mass Simplified Language to handle new use cases (we're experimenting with things like supporting flashloans, intents, etc..)
At the same time, the Mass platform will keep building a great product that will offer a simple UI for the majority of us who don’t want to write code on a daily basis to perform simple actions with our funds.